PowerShell plays a significant role as an administrative scripting language. It is used extensively to manage Active Directory, Microsoft Azure, and Windows Server among many things as well as a tool for developers and automation engineers. Just like any other language, it is vital for scripts to be clean and free of errors for them to be maintainable and secure.
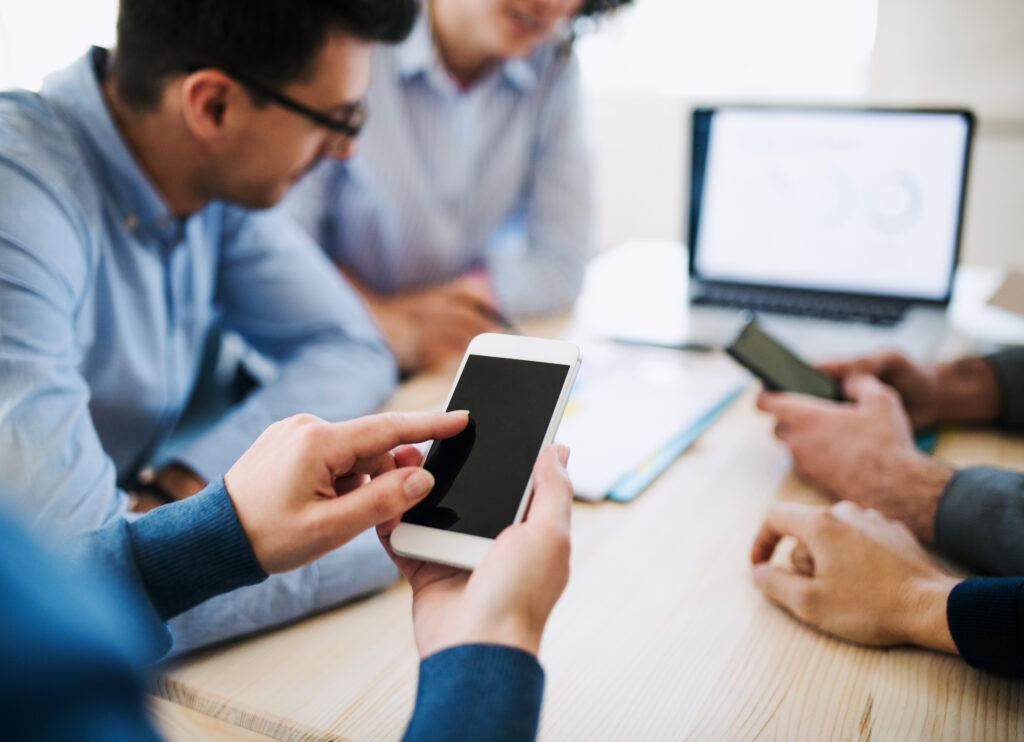
Among the many effective tools such as PoshTools and VS Code which can be used to help write better PowerShell code, there is PSScriptAnalyzer. PSScriptAnalyzer is a static code analysis tool that scans PowerShell scripts and analyzes them to see if they conform to the best practices, styles, and performance. Let’s review what PSScriptAnalyzer is, how it works, and how you can use it to enhance your PowerShell scripts.
What is PSScriptAnalyzer?
Partner with Microsoft experts you can trust
If it’s time to take that first step toward leveling up your organization’s security, get in touch with Ravenswood to start the conversation.
PSScriptAnalyzer is an open-source PowerShell module supported by Microsoft and the PowerShell community. It analyzes your PowerShell code and highlights items that can be improved or corrected. The tool will look for problems in your scripts from syntax errors, code quality and standards, best practices, etc.
Key Features:
- Code Style and Formatting: Checks that your scripts exhibit a standard formatting style as well as following recommended guidelines.
- Best Practices: Analyzes whether your code is written in reasonable and clean PowerShell style and that it also possesses certain security features.
- Security: Detects a potentially dangerous constructs or practices, such as hard-coded credentials or insecure function calls.
- Performance: Offers tips on optimizing the performance of your code.
- Custom Rules: Enables users to set up the rules according to requirements.
By using PSScriptAnalyzer, you can catch common issues early and make your code more readable and maintainable. It can also be integrated into your continuous integration and deployment (CI/CD) pipeline to automate code analysis part of the build process.
Why Use PSScriptAnalyzer?
1. Adherence to PowerShell scripting standards
In development environments, maintaining consistency in code style and structure is crucial, especially when the code is created and supported by more than one person. Inconsistent coding practice can lead to confusion, complexity, and increased potential for bugs. PSScriptAnalyzer helps foster a consistent coding style across your scripts by flagging deviations from best practices.
Coding with a style that you always adhere to will make the code easy to understand for others when someone else has to open a script that they are not familiar with.
PSScriptAnalyzer flags mistakes in code formatting, and it also aids you in creating better, more optimized scripts. It examines your script against best practices to identify areas where you can improve performance and avoid inefficient practices. For example, it can flag Write-Host
statements, recommend using $null
instead of an empty string, or advise you to use singular nouns for functions.
By correcting these problems up front, your applications work better, consume resources more efficiently and, most importantly, are easier to support in the future.
2. Identifies potential issues early
Security is always a priority, especially when it comes to PowerShell scripts that interact with sensitive data or network resources. PSScriptAnalyzer can help detect common security vulnerabilities, such as:
- The use of plain-text credentials
- Executing commands without proper validation
- Allowing for remote execution of scripts without adequate security controls
- For example, you could have a script that requires a password to be passed.
function Get-AllDates {
[CmdletBinding()]
Param(
[string] $timePOS,
[string] $Password
)
# Function logic goes here
}
Running script analyzer returns the following:
RuleName Severity ScriptName Line Message
-------- -------- ---------- ---- -------
PSAvoidUsingPlainTextForPassword Warning Bad-Script 8 Parameter '$Password' should not use String type but either
.ps1 SecureString or PSCredential, otherwise it increases the
chance to expose this sensitive information.
$Password
parameter is using string instead of a SecureString
.
Using PSScriptAnalyzer you can catch potential security risks early and mitigate them before they become a problem. Getting Started
1. Installing PSScriptAnalyzer
Before you can start using PSScriptAnalyzer, you will need to install it. The installation process is easy and straightforward.
To install PSScriptAnalyzer from the PowerShell Gallery, open a PowerShell session and run:
Install-Module -Name PSScriptAnalyzer -Force -Scope CurrentUser
-Force
ensures that you install the latest version even if you have a previous version installed.-Scope CurrentUser
installs the module only for the current user, so you don’t need administrator privileges.
Get-Module -ListAvailable PSScriptAnalyzer
Invoke-ScriptAnalyzer
cmdlet. This cmdlet takes one or more PowerShell scripts as input and performs a static analysis on the code.
For example, if you have a script called myscript.ps1 that you want to analyze, you can run: Invoke-ScriptAnalyzer -Path "C:\path\to\myscript.ps1"
Or if you have several scripts in a folder to analyze, you can run:
Invoke-ScriptAnalyzer -Path "C:\path\to\folder"
The command will return a list of warnings, errors, and suggestions related to the script(s).
3. Understanding the Output
The output from PSScriptAnalyzer will display a list of issues found in the script, along with their severity (e.g., Warning, Error, Information), line number and a description of the issue.
Here’s an example of what the output might look like:
RuleName Severity ScriptName Line Message
-------- -------- ---------- ---- -------
PSUseConsistentWhitespace Warning MyScript.ps1 5 'Operator' should be surrounded by spaces.
PSAvoidUsingWriteHost Warning MyScript.ps1 8 Avoid using Write-Host for output.
PSUseSingularNouns Suggestion MyScript.ps1 12 Use singular nouns for function names.
PSAvoidTrailingWhitespace Information MyScript.ps1 23 Line has trailing whitespace
- RuleName: The name of the rule that was violated.
- Severity: The severity of the issue (e.g., Warning, Error, or Suggestion).
- ScriptName: The script being scanned.
- Line: The line number where the issue occurs.
- Message: A description of the issue.
A full list of the default rules may be found here: List of PSScriptAnalyzer rules – PowerShell | Microsoft Learn
4. Fixing Issues
Once you have identified the issues in your code, it’s time to fix them. The output from PSScriptAnalyzer will guide you on what to change. As an example, if it tells you to “Avoid using Write-Host,” you can replace Write-Host
with Write-Output
or Write-Verbose
depending on your requirements.
5. Using Built-in Presets
Since ScriptAnalyzer has a large set of built-in presets that can be used to analyze scripts, you can run those as well as the defaults. For example, if you want to run PowerShell Gallery rules on your script, use the following command:
Invoke-ScriptAnalyzer -Path "C:to “C:\path\to\myscript.ps1” -Settings PSGallery
You can use tab completion to see all the settings parameters available.
6. Excluding rules
Sometimes you may want to exclude various rules as they may not apply to the script you are creating. As an example, your script may make use of Write-Host and the colorizing of text to the screen. Normally the analyzer would flag the use of Write-Host and create noisy output. You can suppress the output for that rule, and any other rule as well, by using the -ExcludeRule parameter like so:
Invoke-ScriptAnalyzer -Path "Path\To\Your\Script.ps1“ -ExcludeRule PSAvoidUsingWriteHost
-CustomRulePath
parameter to provide a settings file that contains custom rules. For example: Invoke-ScriptAnalyzer -Path "C:to “C:\path\to\myscript.ps1” -CustomRulePath “C:\path\to\custom_rules.psd1”
You can learn more about creating and using custom rules here: Creating custom rules – PowerShell | Microsoft Learn
Tips on Using PSScriptAnalyzer in Your Process
1. In Visual Studio Code, you can use PSScriptAnalyzer directly.
If you are using Visual Studio code (VSCode) for your PowerShell development, then PSScriptAnalyzer can be loaded directly into the code editor. To do this, install the PowerShell extension for VSCode and PSScriptAnalyzer will run on any script you have open within VSCode providing inline linting as you are writing.
2. PSScriptAnalyzer integration in CI/CD Pipelines.
For teams using continuous integration/continuous deployment (CI/CD) pipelines, you can add PSScriptAnalyzer to your pipeline to automate code analysis. For example, in Azure DevOps or GitHub Actions, you can use a PowerShell task to run Invoke-ScriptAnalyzer as part of the build process. This ensures that all code is analyzed before being merged or deployed meaning that all code is checked before being accepted into the main branch or going live.
Best Practices for Using PSScriptAnalyzer
- Run it frequently: Using PSScriptAnalyzer regularly during development will help discover issues before they turn into problems.
- Review output carefully: Take the time to read the output and understand why certain issues are flagged. Some warnings might be subjective or related to team preferences.
- Customize your rules: Customize PSScriptAnalyzer to fit your team’s coding standards. You may need to disable some rules that don’t apply to your specific case or enforce stricter rules for consistency.
- Integrate into your workflow: Integrate PSScriptAnalyzer in your development environment and within the CI/CD pipeline to validate that your scripts are in the best shape that they can be.
Conclusion
PSScriptAnalyzer is a great module for any PowerShell developer wanting to write scripts that are cleaner, more efficient, and more secure. If you work alone or together with a team, using PSScriptAnalyzer can ensure compliance with standard coding practices, help reduce errors, and enhance the quality of the product.
Thanks to its simplicity, flexible rules that can be set and adapted by the user, and integration with Microsoft VSCode, Git, and various CI/CD pipelines, PSScriptAnalyzer is a tool that will help anyone who wants to write clean, solid PowerShell code.
Need guidance on managing your AD and Azure environments? Contact the experts at Ravenswood Technology today. We’re here to help!